Python Meets iOS: Run AI Functions Directly in Your App
Introducing Function for Swift.
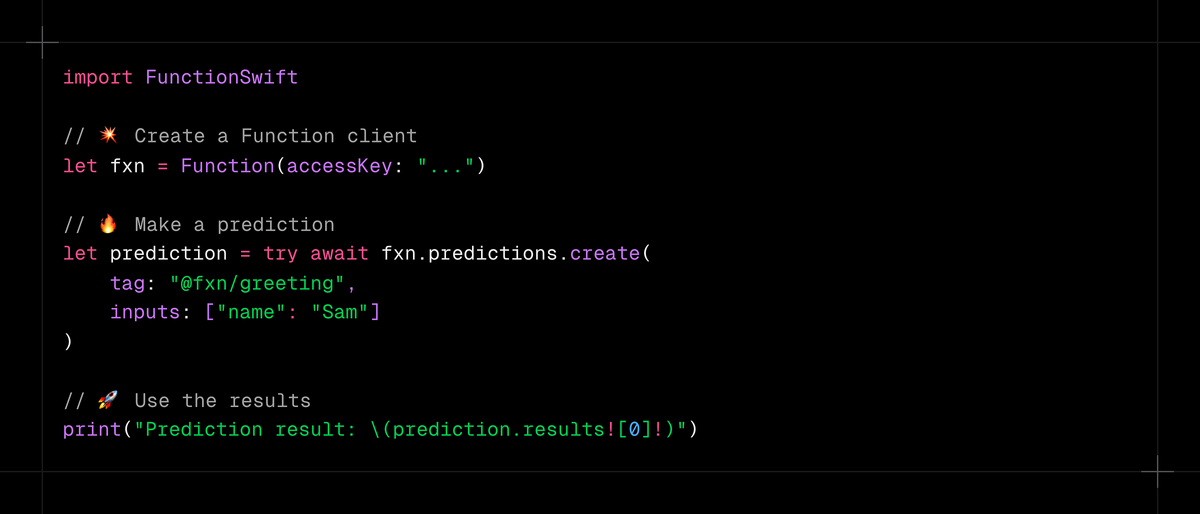
AI is now showing up everywhere, but for a team that wants to integrate an AI model into their iOS app, they face an extremely difficult process: First they have to find an open-source model; try to convert it to CoreML (no guarantees it’ll work); then write a ton of Swift scaffolding to properly feed the model and make sense of its outputs. What if running any open-source AI model on-device was as easy as calling a function? We’re introducing Function for Swift to do just that.
Two Lines of Code
As you saw at the very top of this article, Function enables you to run stateless Python functions in your iOS app in as little as two lines of code:
import FunctionSwift
// 💥 Create a Function client
let fxn = Function(accessKey: "...")
// 🔥 Make a prediction
let prediction = try await fxn.predictions.create(
tag: "@fxn/greeting",
inputs: ["name": "Sam"]
)
// 🚀 Use the results
print("Prediction result: \(prediction.results![0]!)")
We are building a library of open-source, commercial-use prediction functions that developers and enterprises can discover and quickly integrate into their applications. These functions will include everything from text generation with LLMs, to image processing, and anything else that can be squeezed into a stateless Python function.
How it Works
At its core, Function is a Python compiler. A developer provides us with a pure function or functor:
from fxn import compile
@compile(
tag="@fxn/greeting",
description="Say a friendly greeting!"
)
def greeting (name: str) -> str:
return f"Hey there {name}! We're glad you're trying out Function and we hope you like it 😉"
And our platform uses a symbolic tracing and static analysis to transform their code into C++:
std::string predictor (const std::string& name) {
std::string format = "Hey there {}! We're glad you're trying out Function and we hope you like it 😉";
return format_op(format, name);
}
We compile this C++ code to run natively across Android, iOS, macOS, Linux, Windows, and the browser (using WebAssembly). At runtime, our client library simply downloads the compiled binary and executes it.
Try it Out
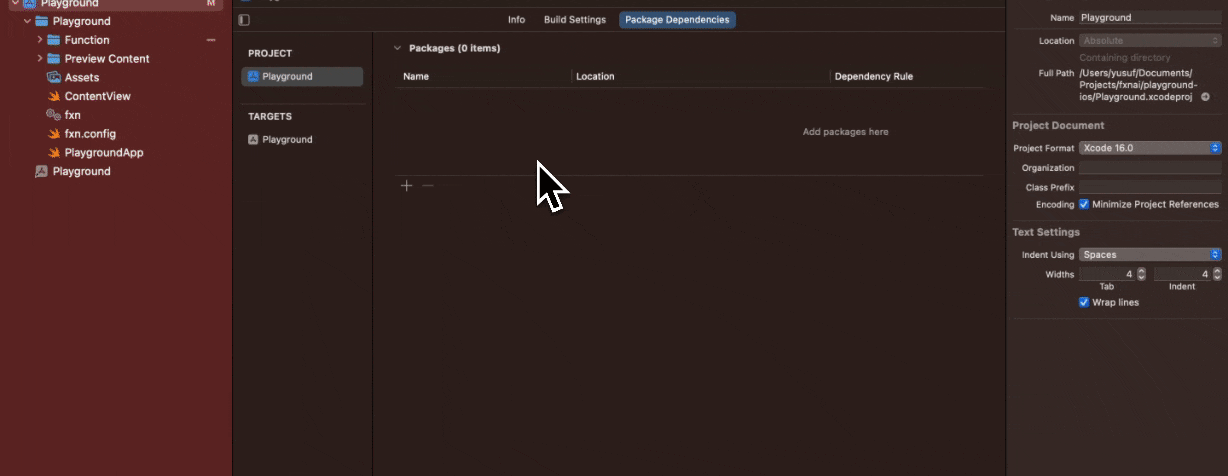
Add Function for Swift to your Xcode project, and follow our docs to make your first prediction. Then head over to Function to explore our current catalog of AI models (we’ve got many more coming!):
If you are deploying on-device AI pipelines in your iOS app, reach out to us at stdin@fxn.ai